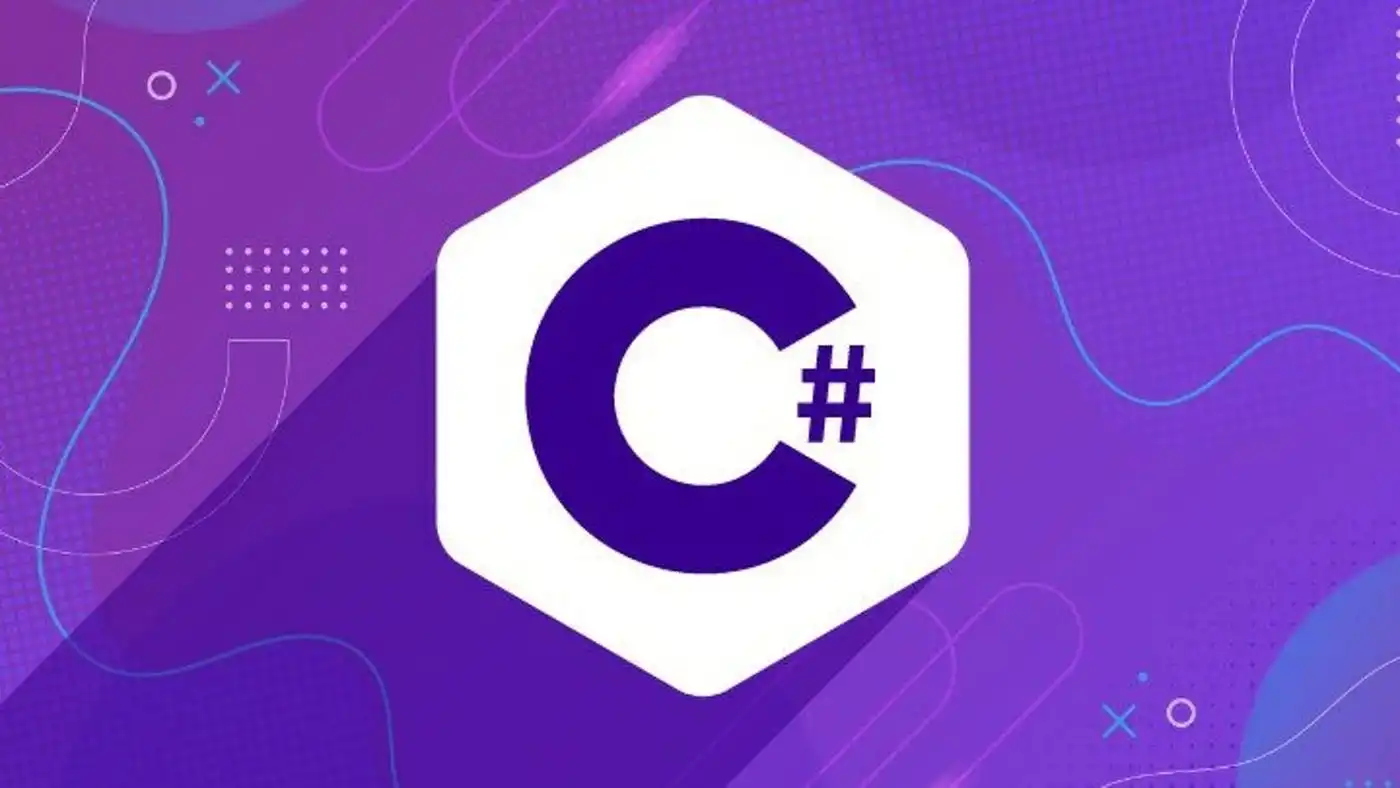
C# : 인덱서(Indexer)
public string this[int index]
{
get { return (index % 2 == 0) ? "짝수" : "홀수"; }
}
인덱서는 클래스의 인스턴스를 배열처럼 사용할 수 있게 하는 구문이다.
쉽게 말해서, 인스턴스 이름 옆에 [index] 붙이면 무슨 행동을 할지 정의하는 메서드이다.
아래의 코드를 보면 조금 더 이해가 잘 될 것이다.
public class Person
{
public string Name { get; set; }
public string Gender { get; set; }
public int Age { get; set; }
public string Email { get; set; }
public Object this [int index]
{
get
{
if (index == 0)
return Name;
else if (index == 1)
return Gender;
else if (index == 2)
return Age;
else if (index == 3)
return Email;
else
return null;
}
set
{
if (index == 0)
Name = value.ToString();
else if (index == 1)
Gender = value.ToString();
else if (index == 2)
Age = Convert.ToInt32(value);
else if (index == 3)
Email = value.ToString();
}
}
public override string ToString()
{
return "Name: " + Name + " / Gender: " + Gender + " / Age: " + Age + " / Email: " + Email;
}
}
class Program
{
static void Main(string[] args)
{
Person person = new Person() {
Name="Tom",
Gender="Male",
Age= 20,
Email="Tom@Test.com"
};
Console.WriteLine("Name: " + person[0]);
Console.WriteLine("Gender: " + person[1]);
Console.WriteLine("Age: " + person[2]);
Console.WriteLine("Email: " + person[3]);
}
}
코드 출처: https://developer-talk.tistory.com/323
person[0]은 Name를 반환하고,
person[1]은 Gender를 반환한다.
이렇게 멤버에 인덱싱을 해줄 수 있는게 바로 인덱서다.
(숫자 말고 문자열로 인덱싱을 해줄 수도 있다.)